First published on
chrishutchinson.me/journal/entry/hacking-around-with-javascript-and-shortcuts-in-ios
Hacking around with JavaScript and Shortcuts in iOS 12
Hacking around with JavaScript and Shortcuts in iOS 12
The launch of iOS 12 brought the release of Shortcuts, a first-party app that allows you to create custom workflows for use by Siri, in the share sheet, and in many other places across iOS.
One feature of Shortcuts is the action “Run JavaScript on Web Page”, which allows you to write any arbitrary JavaScript (based on the feature-set supported by Safari on iOS) and run it on the page. Many of you are likely thinking exactly what I did when I first saw this:
Hey! That’s a bit like a Chrome Extension!
In almost every way, what’s provided by Shortcuts is considerably more rudimentary than a Chrome or Firefox extension, but in this post I’ll run through a few Shortcuts of my own that I’ve written (including links to the code), and hopefully demonstrate how this may just been the beginning of something quite powerful.
Note: This post will include step-by-step guides on creating your own JavaScript-powered Shortcuts. Make sure you’ve downloaded the Shortcuts app for iOS first — link here!
Let’s start simple, you may remember the “Do a barrel roll” easter egg from 2011. We’re going to go ahead an implement this as a Shortcut that you can fire on any web page, directly from the iOS native share sheet.
Don’t want to wait? I’ve already built and uploaded this Shortcut to GitHub, so you can download and use it straight away, here.
To start, we’re going to create a new Shortcut, by tapping the + button in the Shortcuts app. Next, use the search box at the bottom to find “Run JavaScript on Web Page”, and add it to your Shortcut. It’ll bring some sample JavaScript, you’re going to want to replace that with the following lines of code (or grab it from GitHub here) to get that barrel roll goodness.
This code is pretty straightforward, but if you’re not familiar with JavaScript:
- Create a <style> tag
- Fill the tag with our barrel-roll 360 degree CSS animation
- Add the <style> tag to the page, triggering the animation
- Call Shortcut’s completion() method, to tell it our code has finished running
Once you’ve got that code added, tap the settings menu button in the top right, and do the following:
- Give your Shortcut a name, try: Do a barrel roll!
- Tick the “Show in Share Sheet” option
- Open the “Accepted Types” menu, and tap “Deselect All” in the top right
- Scroll down, and check “Safari web pages”, and return to settings view
- Tap “Done”
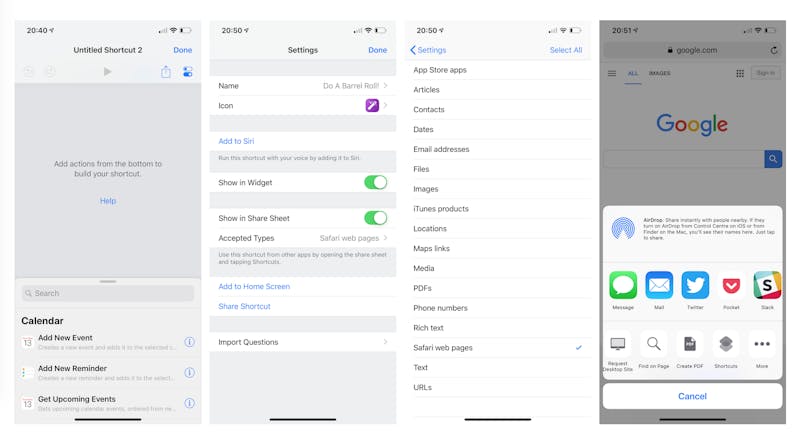
Now your configuration is all set, tap “Done” again to save your Shortcut.
To confirm it works, open Safari, and visit any web page you like. Once the page has loaded, tap the share icon, scroll across to “Shortcuts”, and select your new “Do a barrel roll!” shortcut. Note: the first time you run it, iOS will confirm if you’re happy to run this Shortcut on the website you’ve loaded, you’ll need to confirm. You can see a demo video here.
And there you have it, a pretty simple Shortcut, but one that opens the door for many more possibilities.
Let’s ramp it up a little more, and try to implement a shortcut that activates gyroscope scrolling on any webpage. If your phone is tilted back, it’ll scroll up, start bringing it upright, we’ll scroll down, and bring it up to 90 degrees, and we’ll scroll down even faster.
Don’t want to wait? I’ve already built and uploaded this Shortcut to GitHub, so you can download and use it straight away, here.
Follow the same procedure as before to create a new Shortcut, but paste in the above JavaScript code. This code is a little more complex, but nothing we shouldn’t be able to follow:
- Create a function that handles device orientation change
- Inside that function, grab the “beta” direction (horizontal tilt) and check the value
- Based on the value, do nothing, scroll up, scroll down, or scroll down faster
- Assign our function to the deviceorientation event
- Call the completion() handler to tell Shortcuts we’re done
This is pretty crude, but will do the trick for now. Make sure you set up the share sheet and Safari Web Page triggers in the Shortcut configuration, save it, and give it a whirl. You should be able to tilt your phone back and forth, and scroll up and down the page!
So this is pretty cool, we can interact with the page, get device details, and make it do stuff! You can see a demo video here.
Okay, final demo, this time we’re going to write a script that swaps out words for their emoji equivalent. For the purposes of the test, I’ll provide a small object of words and emojis, but there’s no reason you couldn’t bring in a library (just render out a <script> tag to an external URL or use npm and bundle up your Shortcut code using something like Webpack or Parcel).
Don’t want to wait? I’ve already built and uploaded this Shortcut to GitHub, so you can download and use it straight away, here.
In summary:
- Define a list of words and emojis
- Create a function to replace all the words with their equivalent emojis in a string
- Take the HTML of the page, run it through the function, and set it back again
- Call completion() to tell Shortcuts we’re done
Set this up, just as before, and try running it on the Stranger Things Wikipedia page. You can see a demo video here.
Of course, you don’t only have to interact with the web page, you could pull some data from the page (say, the URL or some kind of content identifier), combine it with data from another service (say, an analytics platform), and send it all back to the user via a notification. I did exactly this as a prototype for The Times:
TIL you can run a block of JavaScript in any web page via iOS 12 Shortcuts. Here's one I hacked together for @TimesDevelops to load article performance data from our analytics platform 📊 pic.twitter.com/Mh5ZleI6Kk
— Chris Hutchinson (@chrishutchinson) October 12, 2018
To do this, when you call the completion() method at the end of your JavaScript, just pass some data along with it, like so:
// Your JavaScript logic here
const data = "Data to send back to Shortcuts";
completion(data);
Once back in the Shortcuts app, you can then add additional actions using this data, like “Copy to Clipboard” and “Send Notification”, along with plenty of others.
This post has covered just a few examples I’ve thrown together over the past 24 hours, but highlights some of the possibilities this feature enables. From personal workflows to workplace tasks there are a huge number of potential use cases for this technology. By integrating this with all the other features of Shortcuts, this might just be the start of a whole new way for JavaScript developers to build for mobile devices.